Introduction to Proxy Python Requests
When it comes to enhancing data extraction and scraping tasks, proxy Python Requests play a vital role in the programmer’s toolkit. Understanding the importance of leveraging proxy servers with Python Requests is crucial for achieving successful scraping outcomes. This article provides a comprehensive guide to utilizing proxy Python Requests effectively, covering essential strategies and best practices.
Key Strategies Overview:
- Importing the requests package for Python
- Creating a proxies dictionary for HTTP and HTTPS connections
- Implementing rotating proxies to evade bans and rate limits
- Utilizing proxy authentication for secure connections
- Exploring advanced proxy strategies for caching, performance enhancement, and security
- Optimizing proxy usage for efficient data acquisition
- Examining case studies and examples of successful proxy implementation
- Providing resources and further learning for expanding knowledge on proxy usage
Key Takeaways
- Understanding the importance of using proxy servers with Python Requests can enhance data extraction and scraping processes.
- Importing the requests package is the first step in utilizing proxy Python Requests, ensuring seamless integration into Python scripts.
- Creating a proxies dictionary allows for the configuration of HTTP and HTTPS connections, specifying proxy addresses and ports for effective communication.
- Rotating proxies is essential for avoiding bans and rate limits during scraping, requiring the implementation of strategies for seamless proxy rotation.
- Utilizing proxy authentication enhances security by setting up authentication for proxy servers and handling authentication challenges within Python Requests.
- Implementing advanced proxy strategies such as caching data, improving performance in web scraping, and enhancing security provides additional benefits beyond basic proxy usage.
- Optimizing proxy usage involves following best practices for efficient data acquisition, monitoring performance, and troubleshooting common issues.
- Real-world case studies and examples demonstrate successful implementation of proxy Python Requests, providing valuable insights for users.
- Accessing resources and further learning materials, including guides, tutorials, and recommended tools, facilitates deeper understanding and mastery of proxy usage.
Introduction to Proxy Python Requests
Proxy Python Requests play a crucial role in enhancing data extraction processes for programmers. By leveraging proxy servers with Python Requests, developers can significantly improve their scraping endeavors. These proxies enable users to hide their IP addresses, enhancing anonymity and security while accessing online resources.
Understanding the importance of using proxy Python Requests: When conducting data extraction tasks, programmers often encounter challenges such as IP bans, rate limiting, and content blocking. Utilizing proxy Python Requests helps mitigate these issues by allowing users to make requests through different IP addresses. This not only prevents detection but also enables access to geo-restricted or blocked content.
Brief overview of the key strategies to be discussed: In this section, the article will delve into various strategies for optimizing the usage of proxy Python Requests. Topics such as creating a proxies dictionary, rotating proxies, handling authentication, and utilizing proxies for caching and performance improvement will be explored in detail.
Importing Python Requests Package
When working with proxy Python Requests, the first step is to import the necessary packages. This ensures that your Python script can utilize the functionalities provided by these packages for handling HTTP requests.
Installing the requests package
To begin, ensure that you have the requests package installed in your Python environment. If it’s not already installed, you can easily do so using pip, the Python package manager. Simply open your command line interface and execute the following command:
pip install requests
This command will download and install the requests package along with its dependencies, making it available for use in your Python scripts.
Importing the requests module into your Python script
Once the requests package is installed, you can import the requests module into your Python script. This module provides convenient methods for sending HTTP requests and handling responses. Importing the module is straightforward and typically done at the beginning of your script:
import requests
With the requests package imported, you’re ready to start using its features to make HTTP requests, including integrating proxies for various purposes such as data extraction, hiding IP addresses, and successful scraping.
Creating a Proxies Dictionary
Defining proxies for HTTP connections
When utilizing proxy Python Requests for HTTP connections, it’s crucial to define proxies effectively to ensure smooth data extraction. Begin by creating a proxies dictionary that includes the necessary parameters for HTTP connections.
To do this, programmers can use the requests package to initiate a dictionary where the key-value pairs represent the proxy settings. The proxies dictionary should specify the proxy address and port for the HTTP connection.
For example:
{'http': 'http://your_proxy_address:port'}
Defining proxies for HTTPS connections
Similar to HTTP connections, defining proxies for HTTPS connections is essential for seamless scraping with Python Requests. Programmers can extend the proxies dictionary to include settings specifically for HTTPS connections.
The structure remains the same as for HTTP connections:
{'https': 'http://your_proxy_address:port'}
Specifying proxy addresses and ports
When specifying proxy addresses and ports within the proxies dictionary, programmers should ensure accuracy to avoid connection errors. The proxy address should point to the proxy server’s IP, and the port should match the port configured for proxy communication.
By meticulously defining proxies for both HTTP and HTTPS connections, developers can optimize their data extraction process and maximize the effectiveness of proxy Python Requests.
Rotating Proxies for Enhanced Scraping
When it comes to data extraction and successful scraping, rotating proxies play a crucial role in hiding IP addresses and managing access restrictions. Let’s delve into the intricacies of using rotating proxies effectively with Python Requests.
Understanding the Need for Rotating Proxies
One of the primary challenges in web scraping is the risk of IP bans and rate limits imposed by websites. Rotating proxies address this issue by constantly changing IP addresses, making it difficult for websites to detect and block scraping activities. By rotating IPs, developers can distribute requests across multiple residential proxies, mimicking natural user behavior and reducing the likelihood of being detected.
Implementing Rotating Proxies to Avoid Bans and Rate Limits
To implement rotating proxies in Python Requests, developers need to create a proxies dictionary containing HTTP and HTTPS connections. By specifying a pool of proxies, developers can rotate IPs with each request, thereby avoiding bans and rate limits. This dynamic proxy rotation ensures continuous data extraction without interruptions.
Exploring Strategies for Managing Proxy Rotation
Effective management of proxy rotation involves several strategies to optimize scraping efficiency and maintain anonymity. Developers can schedule IP rotations at regular intervals, monitor proxy performance, and switch to alternative proxies if necessary. Additionally, implementing proxy authentication ensures secure access to residential proxies and prevents unauthorized usage.
Utilizing Proxy Authentication
Proxy authentication is crucial for securing your connections and ensuring authorized access to proxy servers. By setting up authentication, you can prevent unauthorized usage of your proxies and maintain control over who can access them.
Setting up authentication for proxy servers
When setting up proxy authentication, you typically need to provide credentials such as a username and password or an IP whitelist. This process varies depending on the proxy provider and the type of authentication supported. Many proxy providers, including 123Proxy, offer options for configuring authentication settings through their dashboard or API.
For example, with 123Proxy’s Unmetered Residential Proxies, users can easily set up authentication using either UserPass or IP Whitelist authentication methods. This ensures that only authorized users can access the proxies, adding an extra layer of security to their web scraping or data extraction activities.
Handling authentication challenges within Python Requests
When using Python Requests with authenticated proxies, you may encounter authentication challenges, especially when making requests to websites that require login credentials. To handle these challenges, you can include the authentication details in your request headers.
Here’s a basic example of how to handle authentication challenges within Python Requests:
import requests
proxy = {
‘http’: ‘http://username:password@proxy-ip:port’,
‘https’: ‘http://username:password@proxy-ip:port’
}response = requests.get(‘https://example.com’, proxies=proxy)
print(response.text)
In this example, replace username, password, proxy-ip, and port with your actual authentication details. This will allow Python Requests to authenticate with the proxy server before making the request to the target website.
By utilizing proxy authentication in Python Requests, programmers can ensure secure and authorized access to proxy servers, enabling them to carry out data extraction tasks efficiently and effectively.
Advanced Proxy Strategies
Implementing Proxy Servers for Caching Data
One advanced strategy for utilizing proxy servers with Python Requests is to implement them for caching data. By caching frequently accessed data locally, requests to the target server can be minimized, reducing both latency and bandwidth usage. This is particularly useful in scenarios where the same data is requested repeatedly, such as in web scraping applications where certain pages or resources are accessed frequently.
Implementing caching proxies involves configuring the proxy server to store copies of responses from the target server. Subsequent requests for the same data can then be served directly from the proxy’s cache, eliminating the need to fetch the data again from the original source. This not only speeds up the data retrieval process but also reduces the load on the target server.
Programmers can leverage caching proxies to optimize their data extraction workflows, ensuring faster and more efficient scraping operations.
Utilizing Proxies for Performance Improvement in Web Scraping
Another advanced strategy is to utilize proxies for performance improvement in web scraping. Proxy servers can help distribute scraping requests across multiple IP addresses, reducing the likelihood of IP bans or rate limits imposed by websites. By rotating through a pool of proxies, programmers can distribute the scraping workload evenly and avoid detection.
Furthermore, using proxies can improve the overall performance of web scraping operations by allowing concurrent connections to multiple target servers. This parallel processing capability can significantly speed up data retrieval, especially when dealing with large volumes of information across multiple sources.
By strategically implementing proxies, programmers can enhance the performance of their web scraping scripts, enabling them to extract data more efficiently and reliably.
Enhancing Security Through the Use of Proxies
Proxies can also play a crucial role in enhancing security during web scraping activities. By routing requests through intermediary servers, proxies help conceal the user’s IP address, making it harder for websites to trace scraping activities back to the source.
Additionally, proxies can provide an extra layer of protection against potential security threats, such as malicious attacks or data breaches. By filtering incoming and outgoing traffic, proxies can detect and block suspicious activity, safeguarding the integrity of the scraping process and the underlying systems.
Furthermore, proxy authentication mechanisms can ensure that only authorized users are granted access to the target servers, preventing unauthorized access or abuse of resources.
By integrating proxies into their web scraping workflows, programmers can mitigate security risks and protect both their data and their infrastructure.
Optimizing Proxy Usage
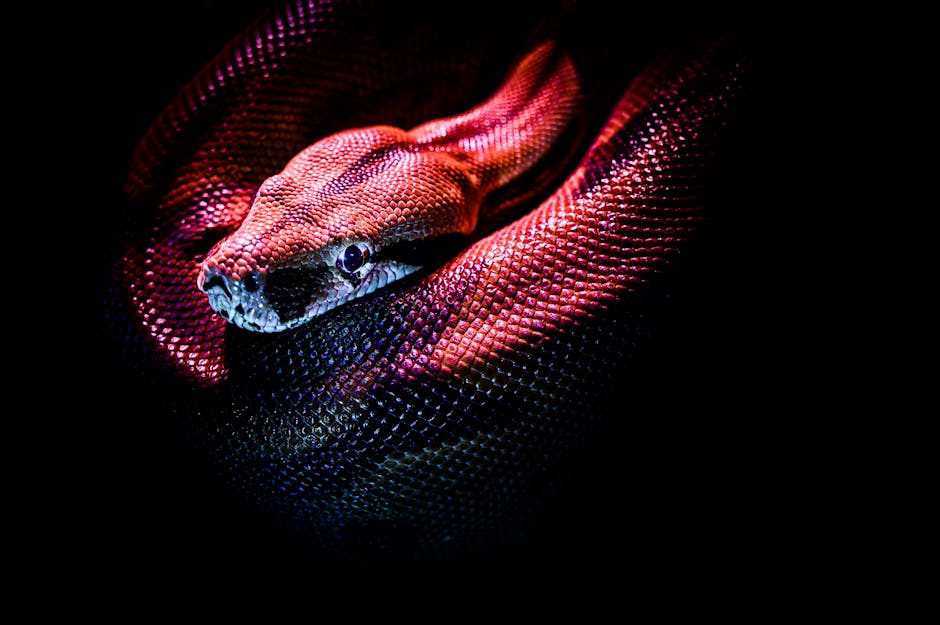
When it comes to optimizing proxy usage for Python Requests, programmers must adhere to best practices to ensure efficient data acquisition while monitoring performance and addressing common issues. Here are key strategies:
Best Practices for Efficient Data Acquisition
To enhance data extraction and successful scraping, programmers should implement proxy Python Requests in a manner that maximizes efficiency. This involves utilizing the requests package effectively, setting up a proxies dictionary with both HTTP and HTTPS connections, and rotating proxies regularly to avoid bans and rate limits.
By hiding IP addresses and leveraging a diverse range of real residential IPs from providers like 123Proxy’s Unmetered Residential Proxies, programmers can ensure their scraping activities remain undetected and maintain a higher success rate.
Monitoring Proxy Performance and Effectiveness
Programmers should establish monitoring mechanisms to track the performance and effectiveness of their proxies. This includes analyzing response times, success rates, and proxy authentication processes. By regularly evaluating these metrics, programmers can identify any issues promptly and make necessary adjustments to optimize performance.
Troubleshooting Common Issues in Proxy Usage
Despite best efforts, proxy usage may encounter common issues such as connection failures, IP bans, or CAPTCHA challenges. Programmers should be prepared to troubleshoot these issues effectively. This involves implementing retry mechanisms, rotating IP addresses more frequently, or utilizing different proxy providers if necessary.
Additionally, referring to guides and tutorials on proxy usage can provide valuable insights into resolving common challenges and optimizing proxy performance for efficient data acquisition.
Case Studies and Examples
Real-world examples of using proxy Python requests
When it comes to leveraging proxy Python Requests for data extraction, real-world examples serve as invaluable learning resources for programmers. These examples showcase how proxies can be integrated into Python scripts to scrape data from websites efficiently and anonymously.
For instance, a programmer might use proxy Python Requests to gather pricing information from e-commerce websites without being blocked or detected. By rotating proxies and utilizing proxy authentication, they can access data from multiple sources without encountering IP bans or rate limits.
Another common example involves web scraping for market research purposes. Companies often employ proxy Python Requests to collect data on competitor pricing, product availability, and customer reviews. With the right proxy strategy in place, programmers can extract large volumes of data accurately and reliably.
Case studies demonstrating successful implementation of proxy strategies
Case studies provide detailed insights into the successful implementation of proxy strategies in various scenarios. These studies highlight the effectiveness of different strategies for data extraction, hiding IP address, and achieving successful scraping outcomes.
For example, a case study might explore how a marketing firm used proxy Python Requests to collect social media data for audience analysis. By employing rotating proxies and proxy authentication, the firm was able to gather comprehensive insights without being blocked by platform restrictions.
Similarly, a case study focusing on web scraping in the finance industry could demonstrate how proxies were used to aggregate stock market data from multiple sources. Through careful caching and performance improvement strategies, the company optimized its data acquisition process and gained a competitive edge in financial analysis.
Summary
Using proxy Python Requests can significantly enhance data extraction by concealing the user’s IP address and increasing the likelihood of successful scraping. This article has provided a comprehensive guide to leveraging proxies effectively for web scraping purposes.
Starting with an introduction to the importance of using proxy servers with Python Requests, the article delves into key strategies such as importing the requests package, creating a proxies dictionary, and implementing rotating proxies to avoid bans and rate limits.
Furthermore, it covers proxy authentication methods and explores advanced strategies for caching data, improving performance, and enhancing security through proxies. The importance of optimizing proxy usage for efficient data acquisition is also emphasized, along with monitoring performance and troubleshooting common issues.
Real-world case studies and examples illustrate the practical application of proxy Python Requests, showcasing successful implementation of proxy strategies. Additionally, the article provides resources and links to guides, tutorials, and recommended tools for further learning and expanding knowledge on proxy usage.
For programmers seeking to maximize their web scraping capabilities, integrating 123Proxy’s Unmetered Residential Proxies can offer a reliable solution with a 50M+ IP pool, high-quality real residential IPs from 150+ countries, and unlimited traffic. Geo-targeting at the country level, sticky sessions, and unlimited concurrent sessions provide flexibility and reliability for proxy usage.
With the insights and strategies outlined in this article, programmers can harness the power of proxy Python Requests to optimize their data extraction processes effectively.
FAQ
Q: Why is using proxy servers with Python Requests important?
A: Using proxy servers with Python Requests is important because it allows users to hide their IP addresses and increase their chances of extracting data without being blocked or detected.
Q: How do I install the requests package for Python?
A: To install the requests package for Python, you can use pip, a package manager for Python. Simply open your command line interface and enter the command pip install requests
.
Q: What is a proxies dictionary in Python Requests?
A: A proxies dictionary in Python Requests is used to define proxies for HTTP and HTTPS connections. It specifies the proxy addresses and ports to be used for routing traffic.
Q: Why is rotating proxies important for web scraping?
A: Rotating proxies are important for web scraping because they help to avoid IP bans and rate limits by constantly changing the IP address used for requests, making it harder for servers to detect and block scraping activity.
Q: How do I set up authentication for proxy servers in Python Requests?
A: To set up authentication for proxy servers in Python Requests, you can provide the auth
parameter with the appropriate username and password when making requests through the proxy.
Q: What are some advanced proxy strategies for Python Requests?
A: Some advanced proxy strategies for Python Requests include using proxy servers for caching data, improving performance in web scraping by distributing requests, and enhancing security by routing traffic through trusted proxies.
Q: What are the best practices for optimizing proxy usage?
A: Best practices for optimizing proxy usage include monitoring proxy performance, rotating proxies regularly to avoid detection, and troubleshooting common issues such as connection errors or IP bans.
Q: Where can I find real-world examples of using proxy Python requests?
A: You can find real-world examples of using proxy Python requests in online forums, tutorials, and case studies provided by proxy service providers or web scraping communities.
References
[1]- ZenRows
[2]- ScrapingBee
[3]- Bright Data
[4]- Medium
[5]- Apify
[6]- SOAX
[7]- GeeksforGeeks
[8]- 123Proxy